What the Node docs don't tell you about callbacks is, of course, a trick question. Because the official documentation says NOTHING about callbacks, and many
other
introductory
Node
pages are vague about how callbacks actually work.
Which is strange, given that Node was built, in large part, around callbacks.
All code in Node is executed asynchronously -- meaning as soon as Node executes one call, it will execute the next, even if the first function hasn't returned its results yet. A callback provides a way to ensure that any function depending on the results of another function (say a slow one that reads files from a disk), will not continue to execute until the function that it relies on, designated as a callback function, has been completed.
Hence the name "callback" -- Once the callback function has finished, then, and only then, does it return control to the function that called it.
As central of a role that callbacks play in Node, I was surprised to find very little documentation on them. And the documentation that did cover them offered specific examples but not much in the way of explanation.The reason callbacks aren't discussed is because they are not so much a Node thing, but a JavaScript thing (Node was built to run JavaScript on the server).
The Node docs assume you know JavaScript, which, if you were taught it formally, you might have spent some time studying this feature, which sets the stage for functional programming in JavaScript. Functional programming is all about using functions as arguments. It typically only gets covered as an "advanced" JavaScript tutorials though.
If you learned JavaScript from a free online tutorial, such as I did through Codecademy, then you're borked when it comes to callbacks.
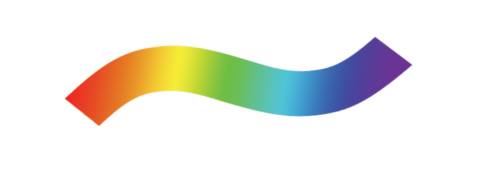
So! Callbacks are functions. And like all functions in JavaScript, callbacks are objects: you can pass them around like any other objects. You can embed functions directly into another function as an argument. See:
var SmoothieMix = ["Banana", "Strawberries", "Milk", "Ice"]; SmoothieMix.forEach (function (ingredient, index){ console.log(index + 1 + ". " + ingredient); });
A callback is not a keyword. It is a placeholder -- the last parameter in a function can be a callback function. Like any other function, we can pass parameters to a callback -- including any of the containing function’s properties.
Here is a callback in action, taken from the JavaScript.Is(Sexy) site.
First, the callback is added to a standard function call:
getUserInput("Michael", "Fassbender", "Man", genericPoemMaker);The name of the function being used as a callback is identified ("genericPoemMaker") as the last value passed to the "getUserInput" function:
function getUserInput(firstName, lastName, gender, callback)when needed, the callback function is summoned within getUserInput and supplied with the necessary arguments:
callback(fullName, gender);And the callback function (genericPoemMaker) completes its task...
function genericPoemMaker(name, gender) { ...snip code... }And when and only when genericPoemMaker is done, it hands control back to getUserInput.
Callback functions can be either anonymous -- simply embedded into the parameter of a function -- or they can be named, such as in the above example.
Boom! That's how callbacks work.